Create your first mashup
To develop a new Mashup, you need to:
- As Administrator, open Targetprocess Settings > Mashups page.
- Press 'Add New Mashup' button
- Select and input placeholder - a place in the Targetprocess UI where your script will be injected and rendered
- Compose source code - the executable body of your mashup
- Save the mashup
- Refresh the page in your web browser with Ctrl+R / Cmd+R.
When a Mashup is saved it is activated for all users in your Targetprocess account. If users have Targetprocess open in their web browser at the moment of saving, then they must refresh the tab manually so that recent changes can be applied to them.
1. Select Placeholder
Every page and view in Targetprocess has a place where you can insert your custom mashup. One of the empty <div> elements with different IDs and class _mashupPlaceholder must be used. Multiple mashups can be attached to the same shared placeholder.
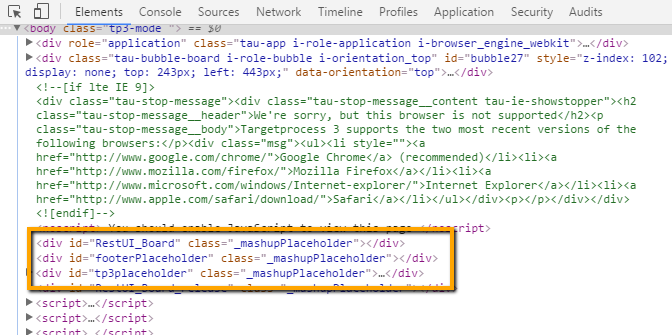
A multipurpose placeholder named footerplaceholder is present in all pages and views. Use it whenever needed. A detailed description of placeholders is available here.
2. Compose Source code
In the executable code you can hook up to a page's structure (DOM) and modify the existing UI. For example, let's create a Mashup that will insert custom block "Custom Block" between "Info" and "Lead Cycle Time" in detailed views for all entities.
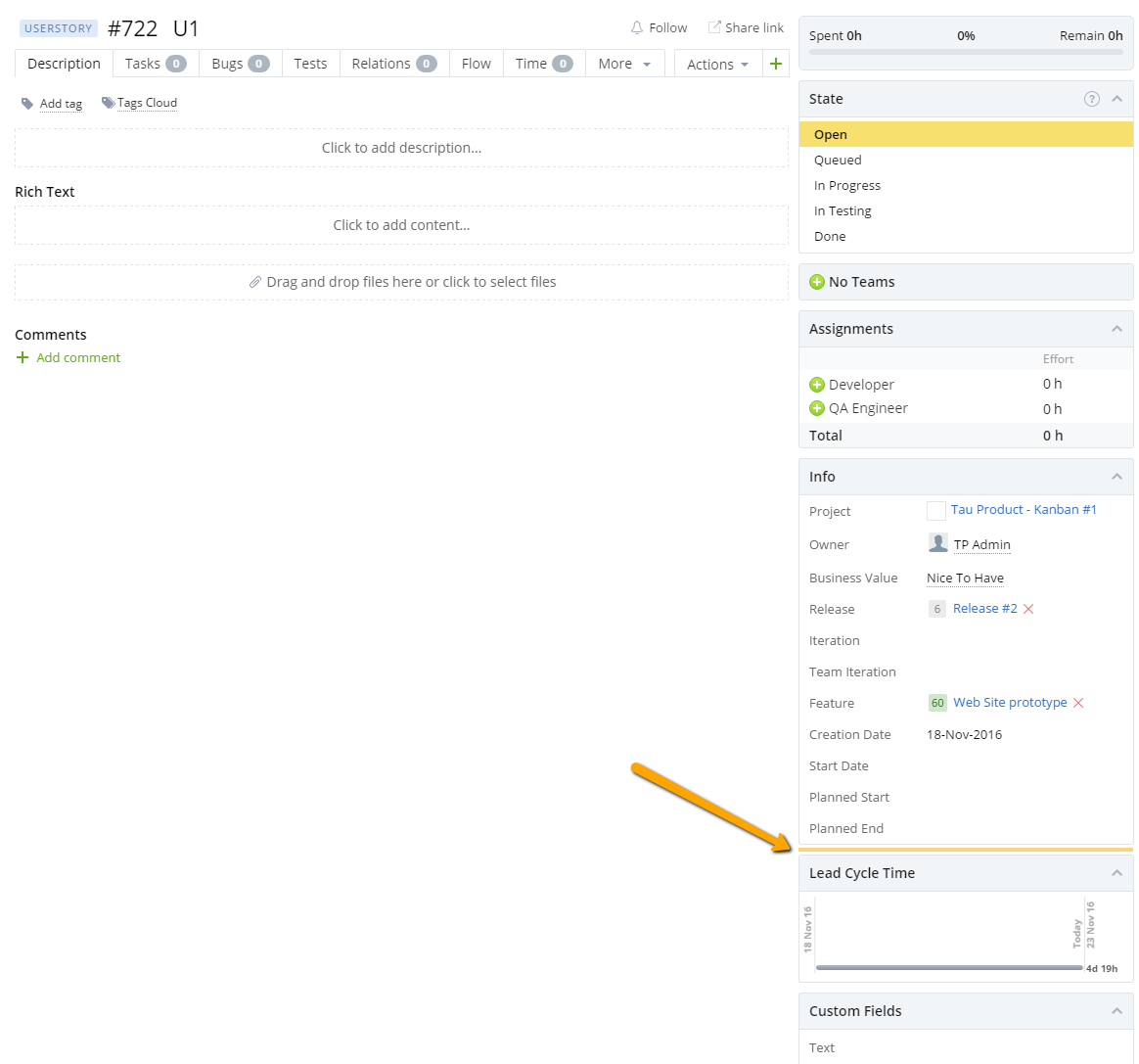
New block will be added here
First, let's build a <div> element for the block with classes and styles already used by existing elements. We can use the Chrome DevTools Elements panel for this purpose. This sample code builds the element:
var newBlockTitle = 'Custom Block';
var newBlockContent = 'Custom Content';
var newBlockContainer = '<div class="tau-container ui-collapsible">' +
'<div class="ui-collapsible-header expanded"><div class="ui-children-container"><div class="ui-label-container"><span class="ui-label">' +
newBlockTitle +
'</span></div></div></div>' +
'<div class="tau-container"><div class="ui-customfield"><table><tbody><tr><td class="ui-customfield__label">' +
newBlockContent +
'</td></tr></tbody></table></div></div>' +
'</div>';
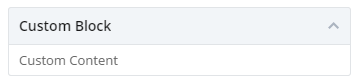
The next part of the code inspects the DOM structure of the loaded view and tries to find a proper place where the new block should be inserted. It uses jQuery's tree traversal functions for this purpose. We terminate execution flow when the page does not contain required place. Furthermore, we'll describe from where $pageElement
resource with all elements of the web page is provided.
var $insertAfterBlockSpan = $pageElement.find('.ui-label:contains("Info")');
var $insertAfterBlockContainer = $insertAfterBlockSpan.closest('.tau-container.ui-collapsible');
if ($insertAfterBlockContainer.length) {
// do something if proper place is found
}
If proper place is found, then our new custom block is appended to the page's structure using jQuery:
$insertAfterBlockContainer.after(newBlockContainer);
The Mashup is almost ready. Now we need to wrap it into a subscription function. It is activated when a page with a detailed view of an entity is rendered by the web browser:
tau.mashups
.addDependency('tp/general/view')
.addMashup(function(view) {
view.onRender(function($pageElement) {
// insert our custom code here
});
});
We are now ready to compose all sample blocks into a single code file.
tau.mashups
.addDependency('tp/general/view')
.addMashup(function(view) {
view.onRender(function($pageElement) {
var newBlockTitle = 'Custom Block';
var newBlockContent = 'Custom Content';
var newBlockContainer = '<div class="tau-container ui-collapsible">' +
'<div class="ui-collapsible-header expanded"><div class="ui-children-container"><div class="ui-label-container"><span class="ui-label">' +
newBlockTitle +
'</span></div></div></div>' +
'<div class="tau-container"><div class="ui-customfield"><table><tbody><tr><td class="ui-customfield__label">' +
newBlockContent +
'</td></tr></tbody> </table></div></div>' +
'</div>';
var $insertAfterBlockSpan = $pageElement.find('.ui-label:contains("Info")');
var $insertAfterBlockContainer = $insertAfterBlockSpan.closest('.tau-container.ui-collapsible');
if ($insertAfterBlockContainer.length) {
$insertAfterBlockContainer.after(newBlockContainer);
}
});
});
3. Save Mashup
Let's install the mashup into your Targetprocess account.
- Login to your account as an Administrator
- Open the Mashup Manager at Settings -> System Settings -> Mashups
- Press the Add New Mashup button
- Type CustomBlockAppender under Name
- Type footerplaceholder under Placeholder(s)
- Copy and paste the whole block of source code under Code
- Press the Save Mashup button
- A green "Mashup has been saved successfully" message should confirm your Save action.
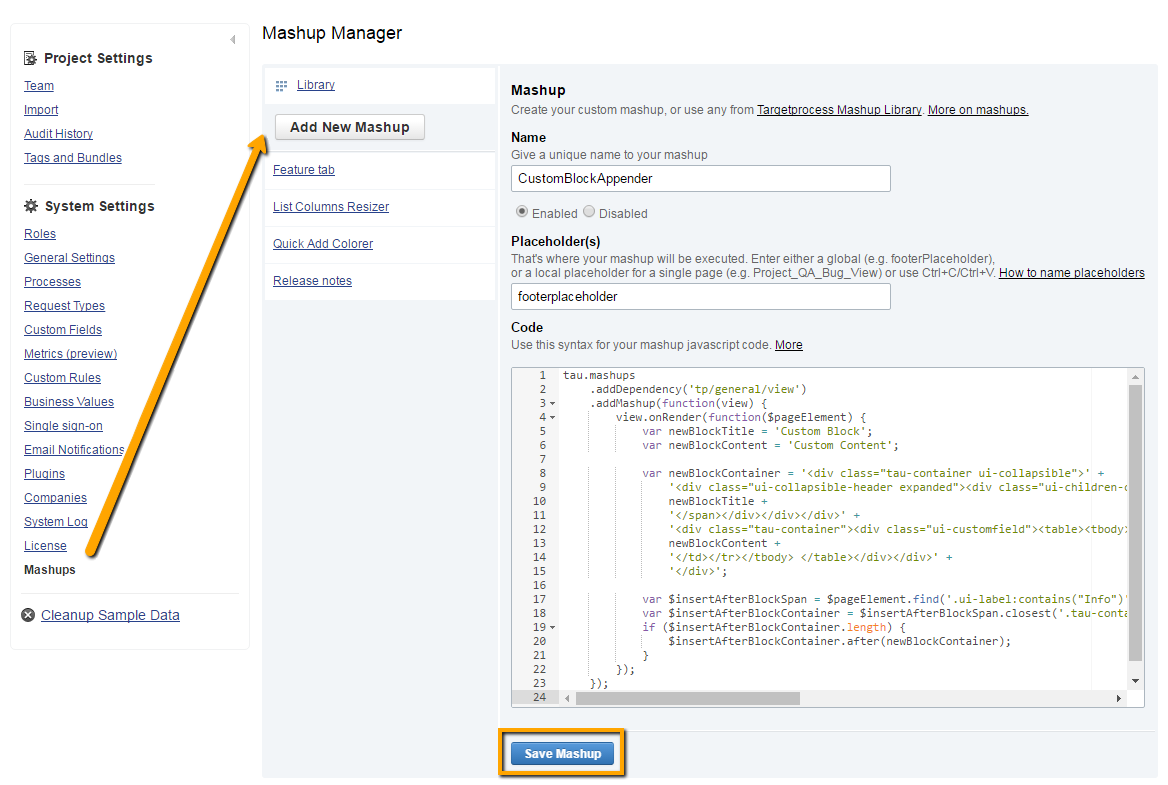
Add and save new mashup
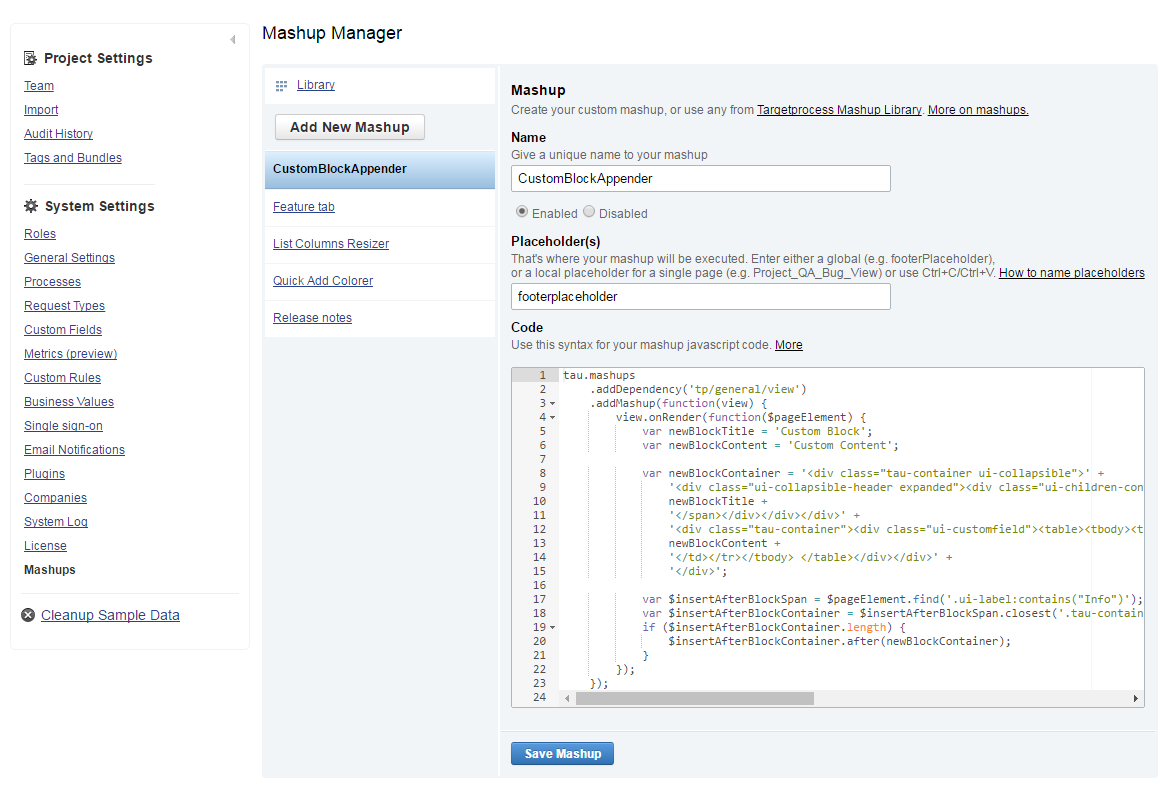
The saved Mashup appears in the list of installed ones
From this list, you can open the settings of your installed Mashup and modify its source code when needed.
4. Check Result
Let's reload a detailed page for a User Story in the web browser. Our Mashup works in detailed views for all entities and all users can see it. We are done!
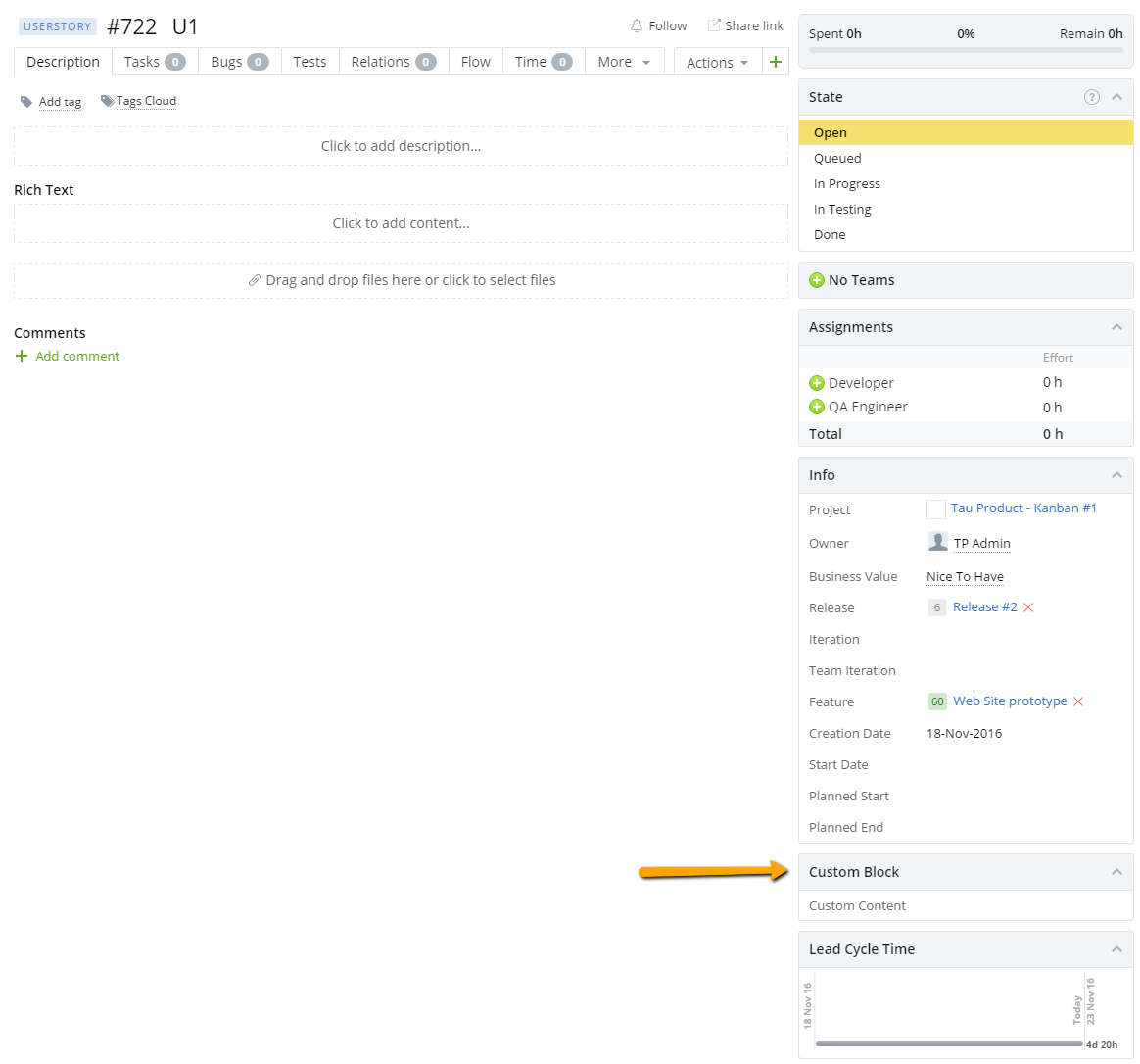
New block is displayed now
Updated about 7 years ago