Start items on their Planned Start date
All of the rules below are almost identical, with the only difference it Entity Type Name in the first line of the code.
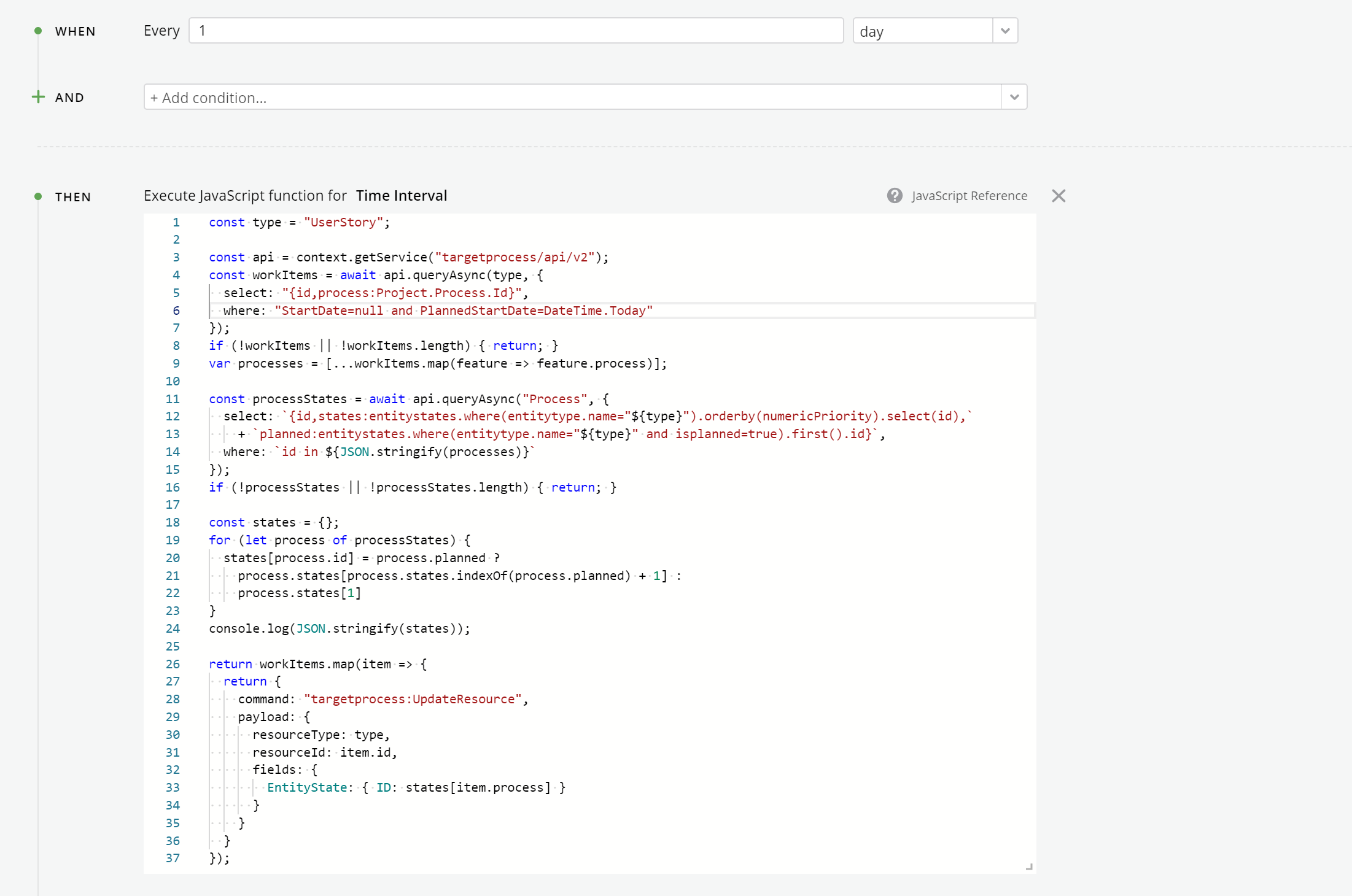
Start User Stories on their Planned Start date
[
{
"type": "source:schedule",
"schedule": {
"kind": "interval",
"unit": "day",
"value": 1
}
},
{
"type": "action:JavaScript",
"script": "const type = \"UserStory\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst workItems = await api.queryAsync(type, {\n select: \"{id,process:Project.Process.Id}\",\n where: \"StartDate=null and PlannedStartDate=DateTime.Today\"\n});\nif (!workItems || !workItems.length) { return; }\nvar processes = [...workItems.map(feature => feature.process)];\n\nconst processStates = await api.queryAsync(\"Process\", {\n select: `{id,states:entitystates.where(entitytype.name=\"${type}\").orderby(numericPriority).select(id),`\n + `planned:entitystates.where(entitytype.name=\"${type}\" and isplanned=true).first().id}`,\n where: `id in ${JSON.stringify(processes)}`\n});\nif (!processStates || !processStates.length) { return; }\n\nconst states = {};\nfor (let process of processStates) {\n states[process.id] = process.planned ?\n process.states[process.states.indexOf(process.planned) + 1] :\n process.states[1]\n}\nconsole.log(JSON.stringify(states));\n\nreturn workItems.map(item => {\n return {\n command: \"targetprocess:UpdateResource\",\n payload: {\n resourceType: type,\n resourceId: item.id,\n fields: {\n EntityState: { ID: states[item.process] }\n }\n }\n }\n});"
}
]
Start Features on their Planned Start date
[
{
"type": "source:schedule",
"schedule": {
"kind": "interval",
"unit": "day",
"value": 1
}
},
{
"type": "action:JavaScript",
"script": "const type = \"Feature\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst workItems = await api.queryAsync(type, {\n select: \"{id,process:Project.Process.Id}\",\n where: \"StartDate=null and PlannedStartDate=DateTime.Today\"\n});\nif (!workItems || !workItems.length) { return; }\nvar processes = [...workItems.map(feature => feature.process)];\n\nconst processStates = await api.queryAsync(\"Process\", {\n select: `{id,states:entitystates.where(entitytype.name=\"${type}\").orderby(numericPriority).select(id),`\n + `planned:entitystates.where(entitytype.name=\"${type}\" and isplanned=true).first().id}`,\n where: `id in ${JSON.stringify(processes)}`\n});\nif (!processStates || !processStates.length) { return; }\n\nconst states = {};\nfor (let process of processStates) {\n states[process.id] = process.planned ?\n process.states[process.states.indexOf(process.planned) + 1] :\n process.states[1]\n}\nconsole.log(JSON.stringify(states));\n\nreturn workItems.map(item => {\n return {\n command: \"targetprocess:UpdateResource\",\n payload: {\n resourceType: type,\n resourceId: item.id,\n fields: {\n EntityState: { ID: states[item.process] }\n }\n }\n }\n});"
}
]
Start Epics on their Planned Start date
[
{
"type": "source:schedule",
"schedule": {
"kind": "interval",
"unit": "day",
"value": 1
}
},
{
"type": "action:JavaScript",
"script": "const type = \"Epic\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst workItems = await api.queryAsync(type, {\n select: \"{id,process:Project.Process.Id}\",\n where: \"StartDate=null and PlannedStartDate=DateTime.Today\"\n});\nif (!workItems || !workItems.length) { return; }\nvar processes = [...workItems.map(feature => feature.process)];\n\nconst processStates = await api.queryAsync(\"Process\", {\n select: `{id,states:entitystates.where(entitytype.name=\"${type}\").orderby(numericPriority).select(id),`\n + `planned:entitystates.where(entitytype.name=\"${type}\" and isplanned=true).first().id}`,\n where: `id in ${JSON.stringify(processes)}`\n});\nif (!processStates || !processStates.length) { return; }\n\nconst states = {};\nfor (let process of processStates) {\n states[process.id] = process.planned ?\n process.states[process.states.indexOf(process.planned) + 1] :\n process.states[1]\n}\nconsole.log(JSON.stringify(states));\n\nreturn workItems.map(item => {\n return {\n command: \"targetprocess:UpdateResource\",\n payload: {\n resourceType: type,\n resourceId: item.id,\n fields: {\n EntityState: { ID: states[item.process] }\n }\n }\n }\n});"
}
]
Updated about 5 years ago