Assign users to a parent when they are working on its child
Assign a User to a User Story when he is assigned to a Task
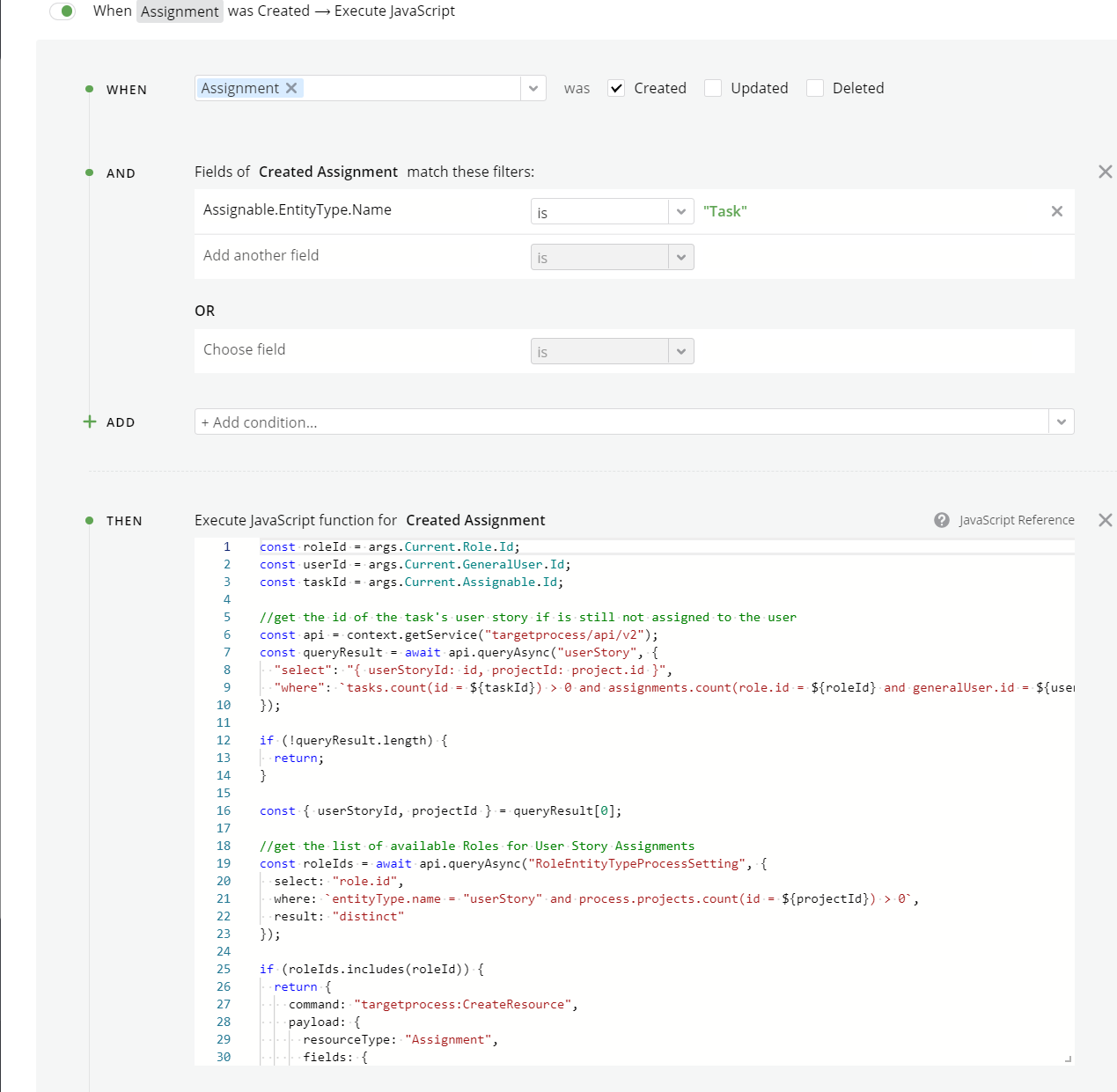
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Assignment"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"or": [
{
"and": [
{
"value": {
"type": "constant",
"value": "Task"
},
"target": {
"name": "Name",
"type": "field",
"target": {
"name": "EntityType",
"type": "field",
"target": {
"name": "Assignable",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"operator": {
"type": "is"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const roleId = args.Current.Role.Id;\nconst userId = args.Current.GeneralUser.Id;\nconst taskId = args.Current.Assignable.Id;\n\n//get the id of the task's user story if is still not assigned to the user\nconst api = context.getService(\"targetprocess/api/v2\");\nconst queryResult = await api.queryAsync(\"userStory\", {\n \"select\": \"{ userStoryId: id, projectId: project.id }\",\n \"where\": `tasks.count(id = ${taskId}) > 0 and assignments.count(role.id = ${roleId} and generalUser.id = ${userId}) = 0`\n});\n\nif (!queryResult.length) {\n return;\n}\n\nconst { userStoryId, projectId } = queryResult[0];\n\n//get the list of available Roles for User Story Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"userStory\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\nif (roleIds.includes(roleId)) {\n return {\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { id: userStoryId },\n Role: { id: roleId },\n GeneralUser: { id: userId }\n }\n }\n }\n}"
}
]
Assign the people working on a Task to its User Story when the User Story is set
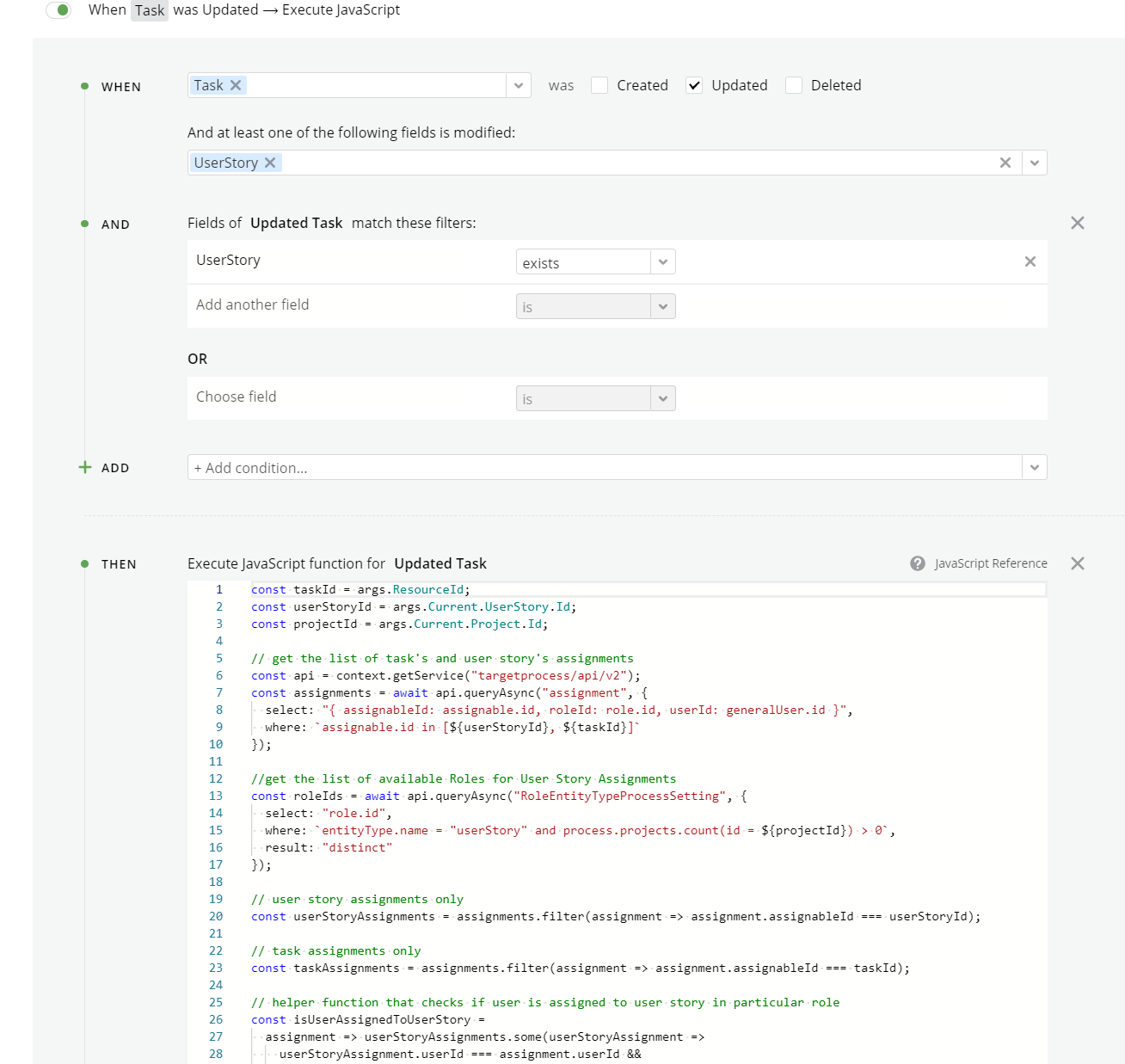
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Task"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"UserStory"
]
}
},
{
"or": [
{
"and": [
{
"value": null,
"target": {
"name": "UserStory",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
},
"operator": {
"type": "exists"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const taskId = args.ResourceId;\nconst userStoryId = args.Current.UserStory.Id;\nconst projectId = args.Current.Project.Id;\n\n// get the list of task's and user story's assignments\nconst api = context.getService(\"targetprocess/api/v2\");\nconst assignments = await api.queryAsync(\"assignment\", {\n select: \"{ assignableId: assignable.id, roleId: role.id, userId: generalUser.id }\",\n where: `assignable.id in [${userStoryId}, ${taskId}]`\n});\n\n//get the list of available Roles for User Story Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"userStory\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\n// user story assignments only\nconst userStoryAssignments = assignments.filter(assignment => assignment.assignableId === userStoryId);\n\n// task assignments only\nconst taskAssignments = assignments.filter(assignment => assignment.assignableId === taskId);\n\n// helper function that checks if user is assigned to user story in particular role\nconst isUserAssignedToUserStory =\n assignment => userStoryAssignments.some(userStoryAssignment =>\n userStoryAssignment.userId === assignment.userId &&\n userStoryAssignment.roleId === assignment.roleId);\n\n// helper function that checks if the role exists in user story workflow\nconst isRoleAvailable =\n assignment => roleIds.some(roleId => assignment.roleId === roleId);\n\n// task assignments that should be reflected for user story\nconst filteredAssignments = taskAssignments.filter(assignment =>\n isRoleAvailable(assignment) && !isUserAssignedToUserStory(assignment));\n\n// create user story assignments\nreturn filteredAssignments.map(assignment => ({\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { Id: userStoryId },\n GeneralUser: { Id: assignment.userId },\n Role: { Id: assignment.roleId }\n }\n }\n}));"
}
]
Assign a User to a Feature when he is assigned to a User Story
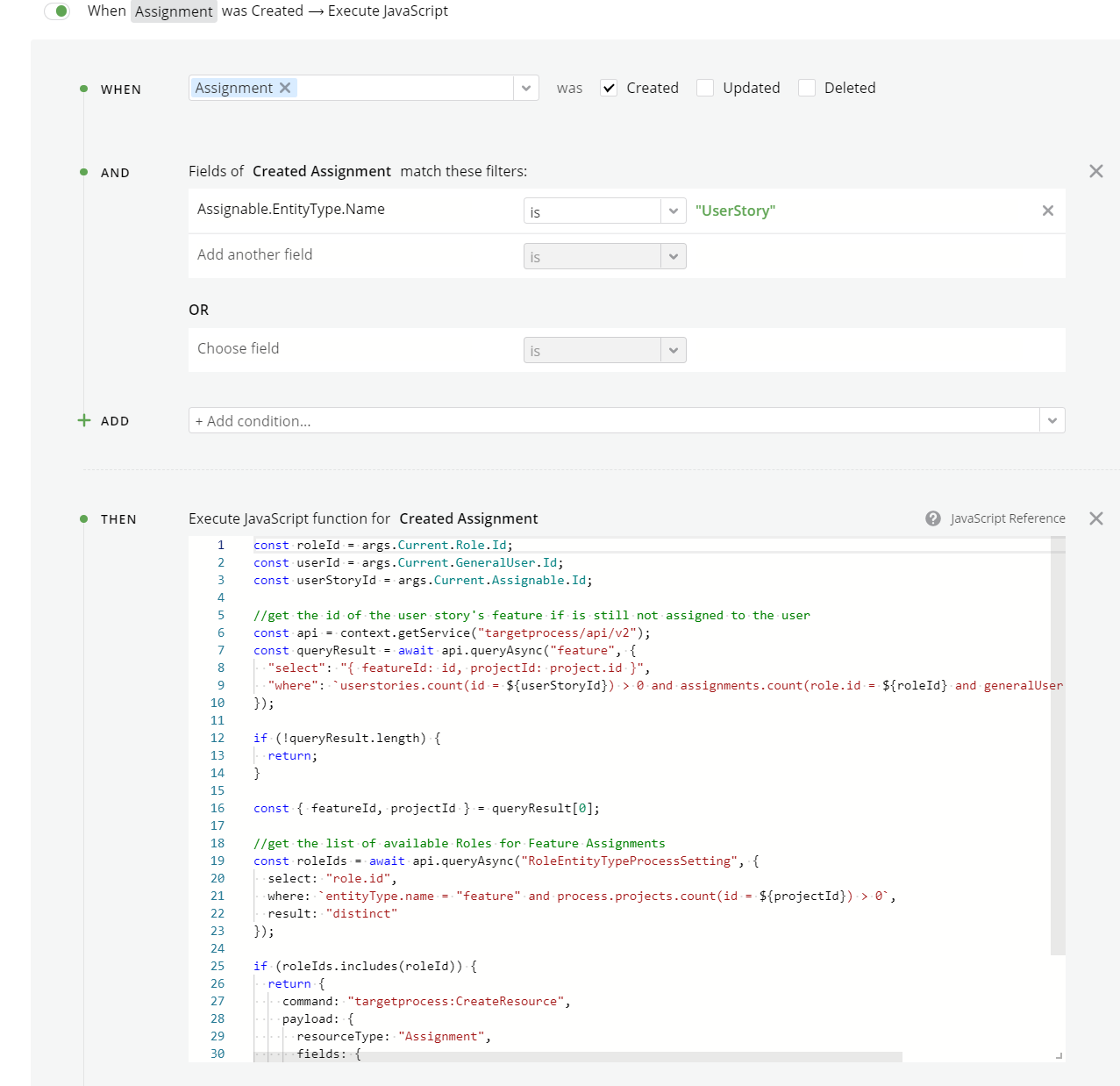
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Assignment"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"or": [
{
"and": [
{
"value": {
"type": "constant",
"value": "UserStory"
},
"target": {
"name": "Name",
"type": "field",
"target": {
"name": "EntityType",
"type": "field",
"target": {
"name": "Assignable",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"operator": {
"type": "is"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const roleId = args.Current.Role.Id;\nconst userId = args.Current.GeneralUser.Id;\nconst userStoryId = args.Current.Assignable.Id;\n\n//get the id of the user story's feature if is still not assigned to the user\nconst api = context.getService(\"targetprocess/api/v2\");\nconst queryResult = await api.queryAsync(\"feature\", {\n \"select\": \"{ featureId: id, projectId: project.id }\",\n \"where\": `userstories.count(id = ${userStoryId}) > 0 and assignments.count(role.id = ${roleId} and generalUser.id = ${userId}) = 0`\n});\n\nif (!queryResult.length) {\n return;\n}\n\nconst { featureId, projectId } = queryResult[0];\n\n//get the list of available Roles for Feature Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"feature\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\nif (roleIds.includes(roleId)) {\n return {\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { id: featureId },\n Role: { id: roleId },\n GeneralUser: { id: userId }\n }\n }\n }\n}"
}
]
Assign the people working on a User Story to its Feature when the Feature is set
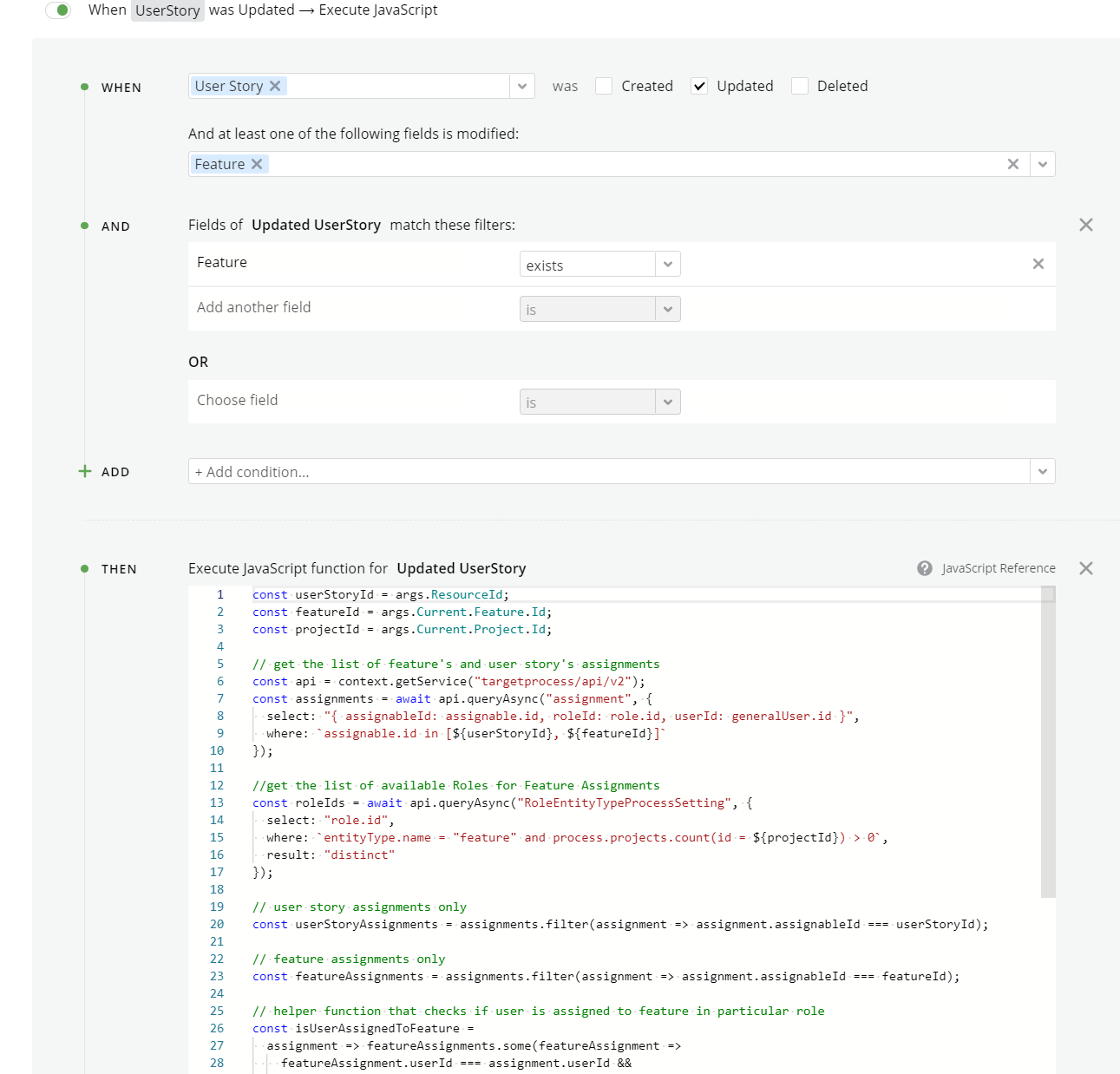
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"Feature"
]
}
},
{
"or": [
{
"and": [
{
"value": null,
"target": {
"name": "Feature",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
},
"operator": {
"type": "exists"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const userStoryId = args.ResourceId;\nconst featureId = args.Current.Feature.Id;\nconst projectId = args.Current.Project.Id;\n\n// get the list of feature's and user story's assignments\nconst api = context.getService(\"targetprocess/api/v2\");\nconst assignments = await api.queryAsync(\"assignment\", {\n select: \"{ assignableId: assignable.id, roleId: role.id, userId: generalUser.id }\",\n where: `assignable.id in [${userStoryId}, ${featureId}]`\n});\n\n//get the list of available Roles for Feature Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"feature\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\n// user story assignments only\nconst userStoryAssignments = assignments.filter(assignment => assignment.assignableId === userStoryId);\n\n// feature assignments only\nconst featureAssignments = assignments.filter(assignment => assignment.assignableId === featureId);\n\n// helper function that checks if user is assigned to feature in particular role\nconst isUserAssignedToFeature =\n assignment => featureAssignments.some(featureAssignment =>\n featureAssignment.userId === assignment.userId &&\n featureAssignment.roleId === assignment.roleId);\n\n// helper function that checks if the role exists in feature workflow\nconst isRoleAvailable =\n assignment => roleIds.some(roleId => assignment.roleId === roleId);\n\n// user story assignments that should be reflected for feature\nconst filteredAssignments = userStoryAssignments.filter(assignment =>\n isRoleAvailable(assignment) && !isUserAssignedToFeature(assignment));\n\n// create feature assignments\nreturn filteredAssignments.map(assignment => ({\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { Id: featureId },\n GeneralUser: { Id: assignment.userId },\n Role: { Id: assignment.roleId }\n }\n }\n}));"
}
]
Assign a User to an Epic when he is assigned to a Feature
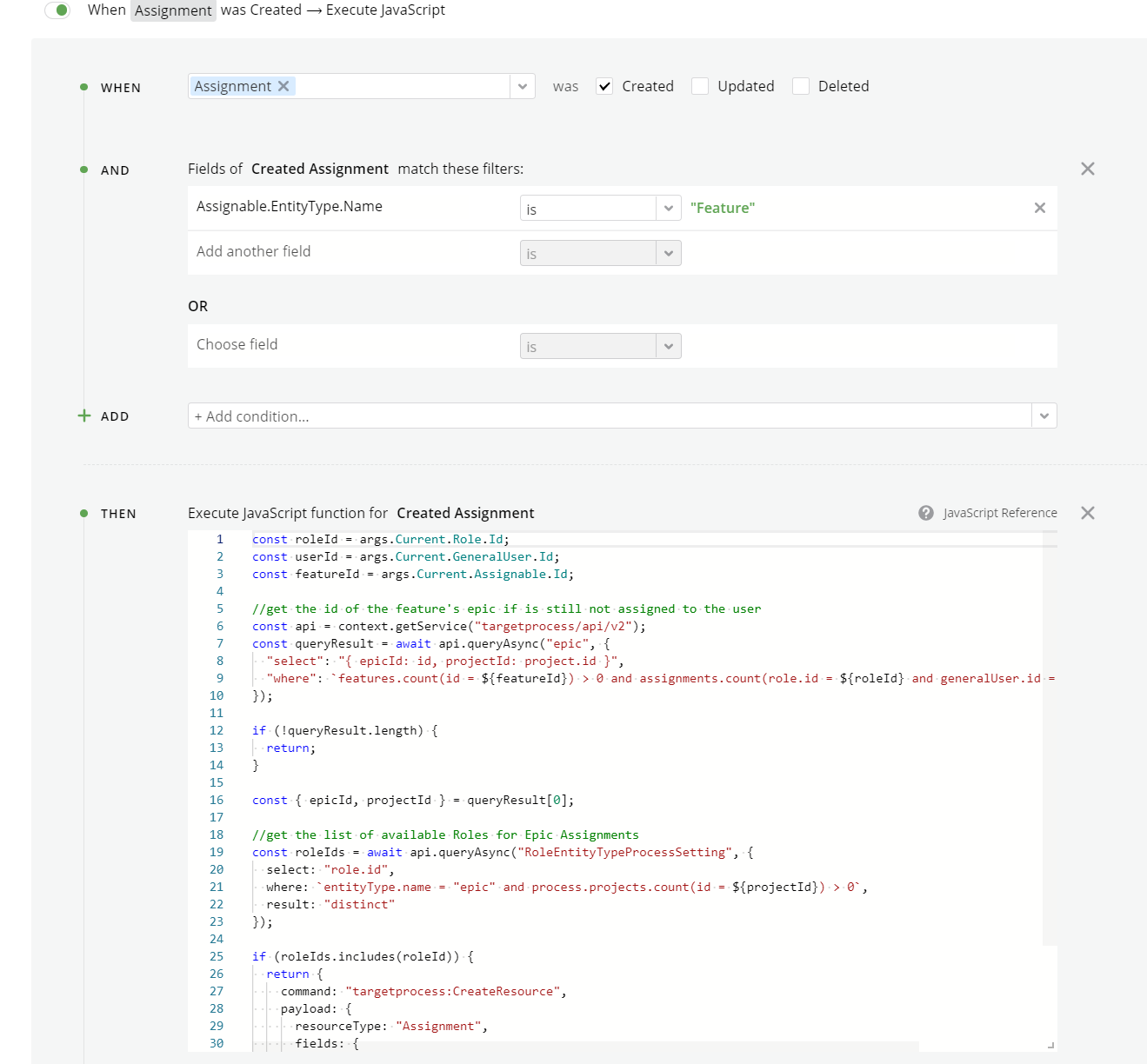
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Assignment"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"or": [
{
"and": [
{
"value": {
"type": "constant",
"value": "Feature"
},
"target": {
"name": "Name",
"type": "field",
"target": {
"name": "EntityType",
"type": "field",
"target": {
"name": "Assignable",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"operator": {
"type": "is"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const roleId = args.Current.Role.Id;\nconst userId = args.Current.GeneralUser.Id;\nconst featureId = args.Current.Assignable.Id;\n\n//get the id of the feature's epic if is still not assigned to the user\nconst api = context.getService(\"targetprocess/api/v2\");\nconst queryResult = await api.queryAsync(\"epic\", {\n \"select\": \"{ epicId: id, projectId: project.id }\",\n \"where\": `features.count(id = ${featureId}) > 0 and assignments.count(role.id = ${roleId} and generalUser.id = ${userId}) = 0`\n});\n\nif (!queryResult.length) {\n return;\n}\n\nconst { epicId, projectId } = queryResult[0];\n\n//get the list of available Roles for Epic Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"epic\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\nif (roleIds.includes(roleId)) {\n return {\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { id: epicId },\n Role: { id: roleId },\n GeneralUser: { id: userId }\n }\n }\n }\n}"
}
]
Assign the people working on a Feature to its Epic when the Epic is set
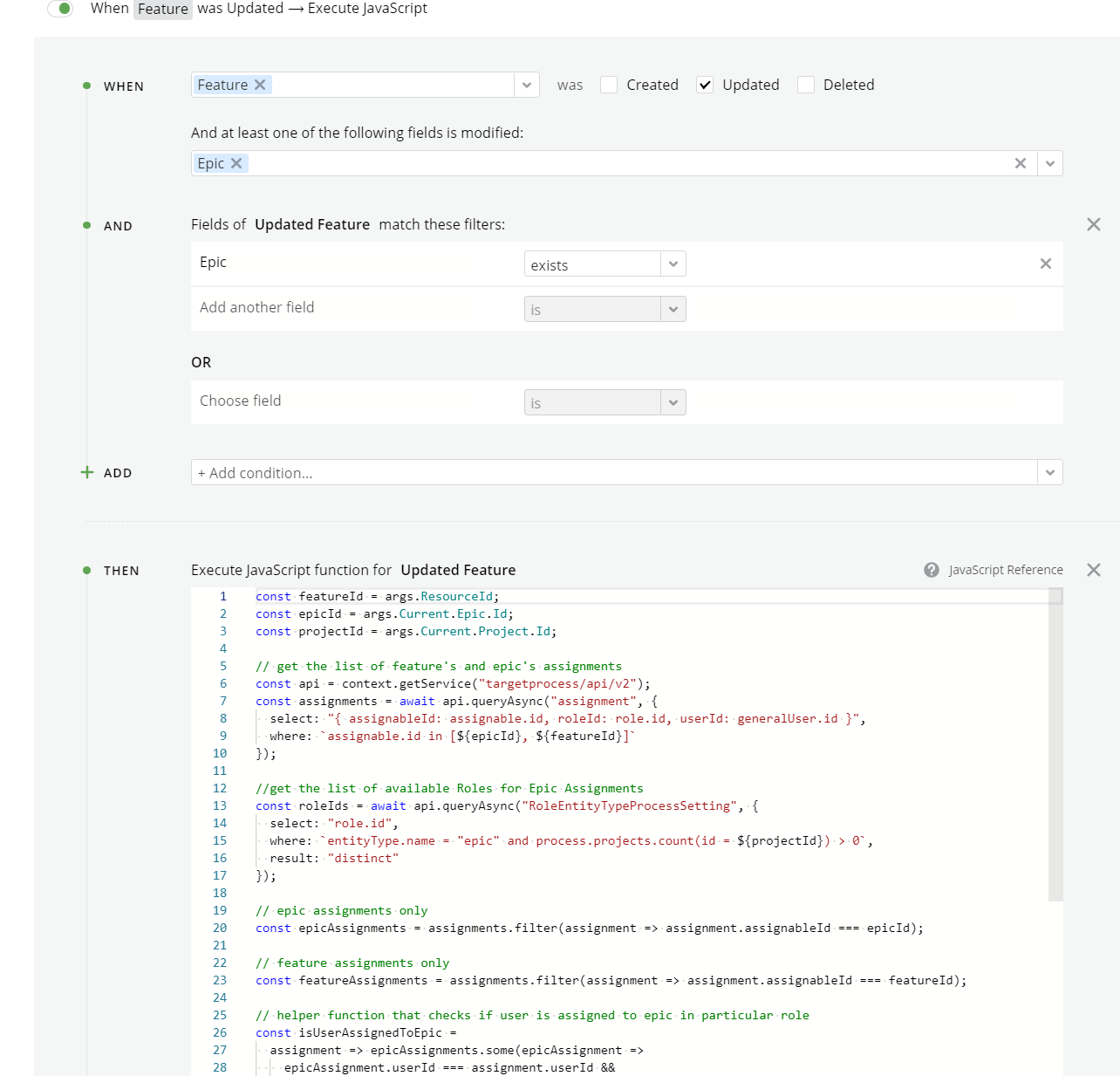
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Feature"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"Epic"
]
}
},
{
"or": [
{
"and": [
{
"value": null,
"target": {
"name": "Epic",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
},
"operator": {
"type": "exists"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const featureId = args.ResourceId;\nconst epicId = args.Current.Epic.Id;\nconst projectId = args.Current.Project.Id;\n\n// get the list of feature's and epic's assignments\nconst api = context.getService(\"targetprocess/api/v2\");\nconst assignments = await api.queryAsync(\"assignment\", {\n select: \"{ assignableId: assignable.id, roleId: role.id, userId: generalUser.id }\",\n where: `assignable.id in [${epicId}, ${featureId}]`\n});\n\n//get the list of available Roles for Epic Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"epic\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\n// epic assignments only\nconst epicAssignments = assignments.filter(assignment => assignment.assignableId === epicId);\n\n// feature assignments only\nconst featureAssignments = assignments.filter(assignment => assignment.assignableId === featureId);\n\n// helper function that checks if user is assigned to epic in particular role\nconst isUserAssignedToEpic =\n assignment => epicAssignments.some(epicAssignment =>\n epicAssignment.userId === assignment.userId &&\n epicAssignment.roleId === assignment.roleId);\n\n// helper function that checks if the role exists in epic workflow\nconst isRoleAvailable =\n assignment => roleIds.some(roleId => assignment.roleId === roleId);\n\n// feature assignments that should be reflected for epic\nconst filteredAssignments = featureAssignments.filter(assignment =>\n isRoleAvailable(assignment) && !isUserAssignedToEpic(assignment));\n\n// create epic assignments\nreturn filteredAssignments.map(assignment => ({\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { Id: epicId },\n GeneralUser: { Id: assignment.userId },\n Role: { Id: assignment.roleId }\n }\n }\n}));"
}
]
Assign a User to a Portfolio Epic when he is assigned to an Epic
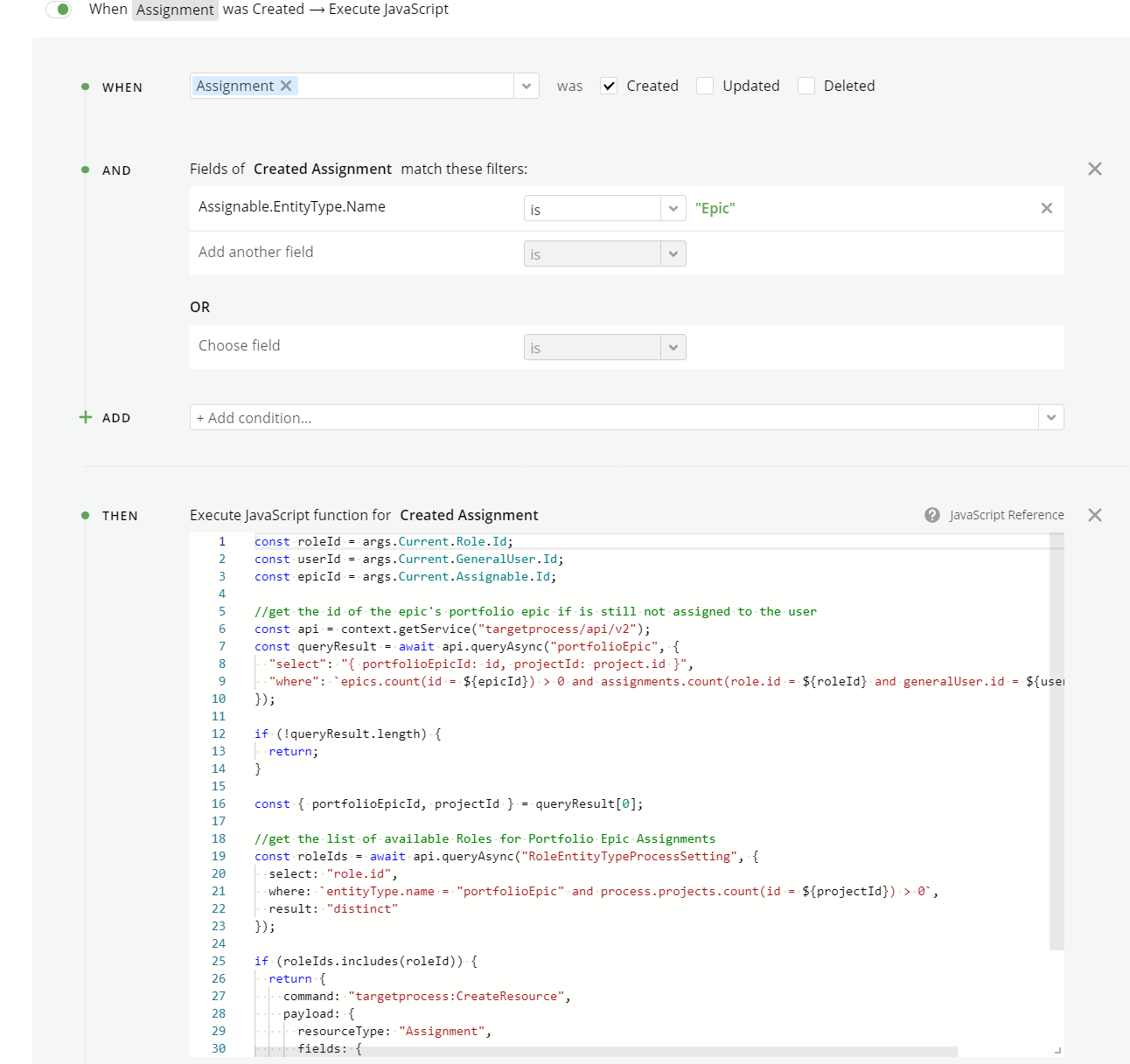
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Assignment"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"or": [
{
"and": [
{
"value": {
"type": "constant",
"value": "Epic"
},
"target": {
"name": "Name",
"type": "field",
"target": {
"name": "EntityType",
"type": "field",
"target": {
"name": "Assignable",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"operator": {
"type": "is"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const roleId = args.Current.Role.Id;\nconst userId = args.Current.GeneralUser.Id;\nconst epicId = args.Current.Assignable.Id;\n\n//get the id of the epic's portfolio epic if is still not assigned to the user\nconst api = context.getService(\"targetprocess/api/v2\");\nconst queryResult = await api.queryAsync(\"portfolioEpic\", {\n \"select\": \"{ portfolioEpicId: id, projectId: project.id }\",\n \"where\": `epics.count(id = ${epicId}) > 0 and assignments.count(role.id = ${roleId} and generalUser.id = ${userId}) = 0`\n});\n\nif (!queryResult.length) {\n return;\n}\n\nconst { portfolioEpicId, projectId } = queryResult[0];\n\n//get the list of available Roles for Portfolio Epic Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"portfolioEpic\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\nif (roleIds.includes(roleId)) {\n return {\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { id: portfolioEpicId },\n Role: { id: roleId },\n GeneralUser: { id: userId }\n }\n }\n }\n}"
}
]
Assign the people working on an Epic to its Portfolio Epic when the Portfolio Epic is set
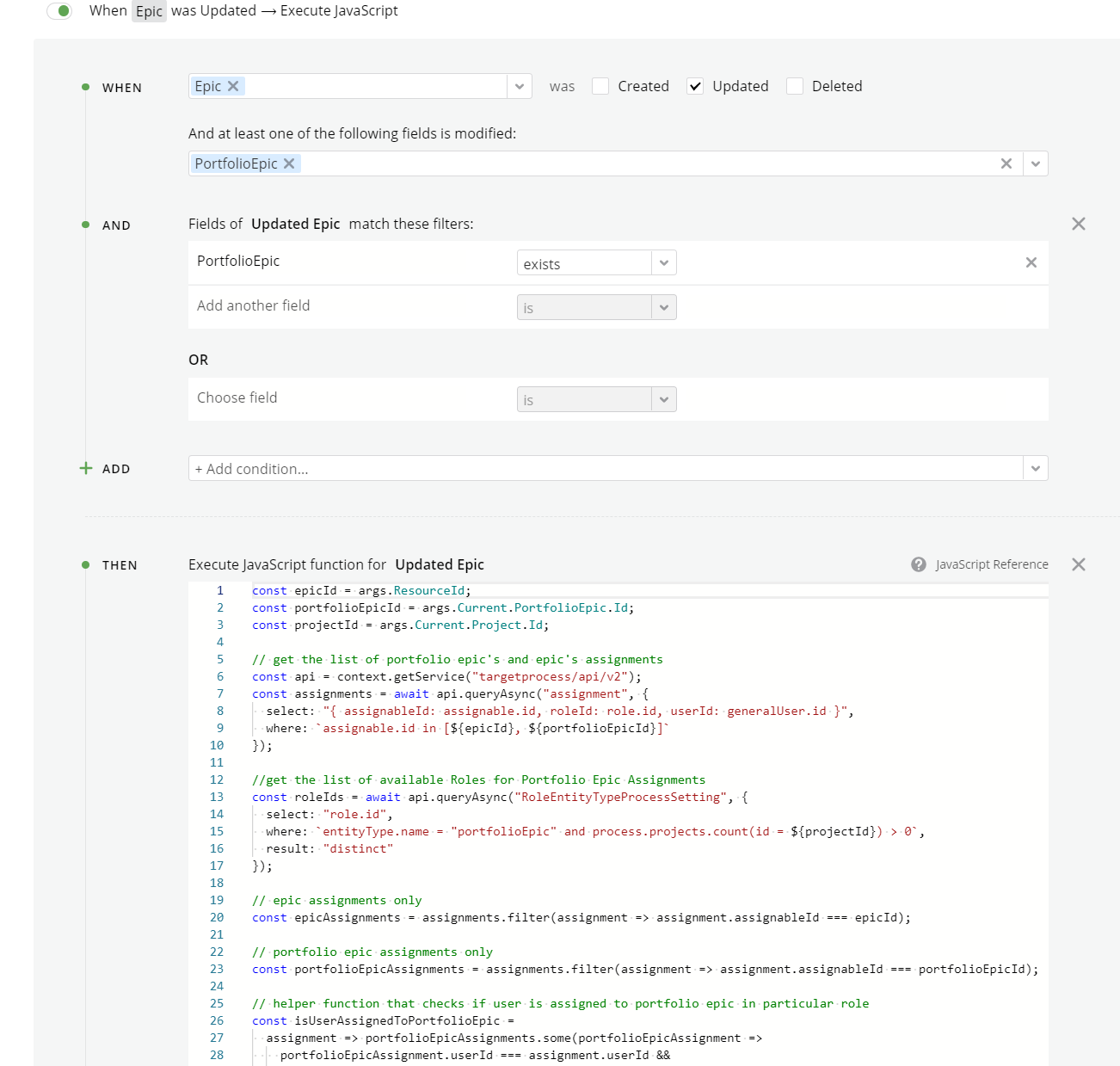
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Epic"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"PortfolioEpic"
]
}
},
{
"or": [
{
"and": [
{
"value": null,
"target": {
"name": "PortfolioEpic",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
},
"operator": {
"type": "exists"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const epicId = args.ResourceId;\nconst portfolioEpicId = args.Current.PortfolioEpic.Id;\nconst projectId = args.Current.Project.Id;\n\n// get the list of portfolio epic's and epic's assignments\nconst api = context.getService(\"targetprocess/api/v2\");\nconst assignments = await api.queryAsync(\"assignment\", {\n select: \"{ assignableId: assignable.id, roleId: role.id, userId: generalUser.id }\",\n where: `assignable.id in [${epicId}, ${portfolioEpicId}]`\n});\n\n//get the list of available Roles for Portfolio Epic Assignments\nconst roleIds = await api.queryAsync(\"RoleEntityTypeProcessSetting\", {\n select: \"role.id\",\n where: `entityType.name = \"portfolioEpic\" and process.projects.count(id = ${projectId}) > 0 and CanBeAssigned = True`,\n result: \"distinct\"\n});\n\n// epic assignments only\nconst epicAssignments = assignments.filter(assignment => assignment.assignableId === epicId);\n\n// portfolio epic assignments only\nconst portfolioEpicAssignments = assignments.filter(assignment => assignment.assignableId === portfolioEpicId);\n\n// helper function that checks if user is assigned to portfolio epic in particular role\nconst isUserAssignedToPortfolioEpic =\n assignment => portfolioEpicAssignments.some(portfolioEpicAssignment =>\n portfolioEpicAssignment.userId === assignment.userId &&\n portfolioEpicAssignment.roleId === assignment.roleId);\n\n// helper function that checks if the role exists in portfolio epic workflow\nconst isRoleAvailable =\n assignment => roleIds.some(roleId => assignment.roleId === roleId);\n\n// epic assignments that should be reflected for epic\nconst filteredAssignments = epicAssignments.filter(assignment =>\n isRoleAvailable(assignment) && !isUserAssignedToPortfolioEpic(assignment));\n\n// create portfolio epic assignments\nreturn filteredAssignments.map(assignment => ({\n command: \"targetprocess:CreateResource\",\n payload: {\n resourceType: \"Assignment\",\n fields: {\n Assignable: { Id: portfolioEpicId },\n GeneralUser: { Id: assignment.userId },\n Role: { Id: assignment.roleId }\n }\n }\n}));"
}
]
Updated over 4 years ago