Close a request when all related items are closed
Close a Request when all related inbound entities are closed
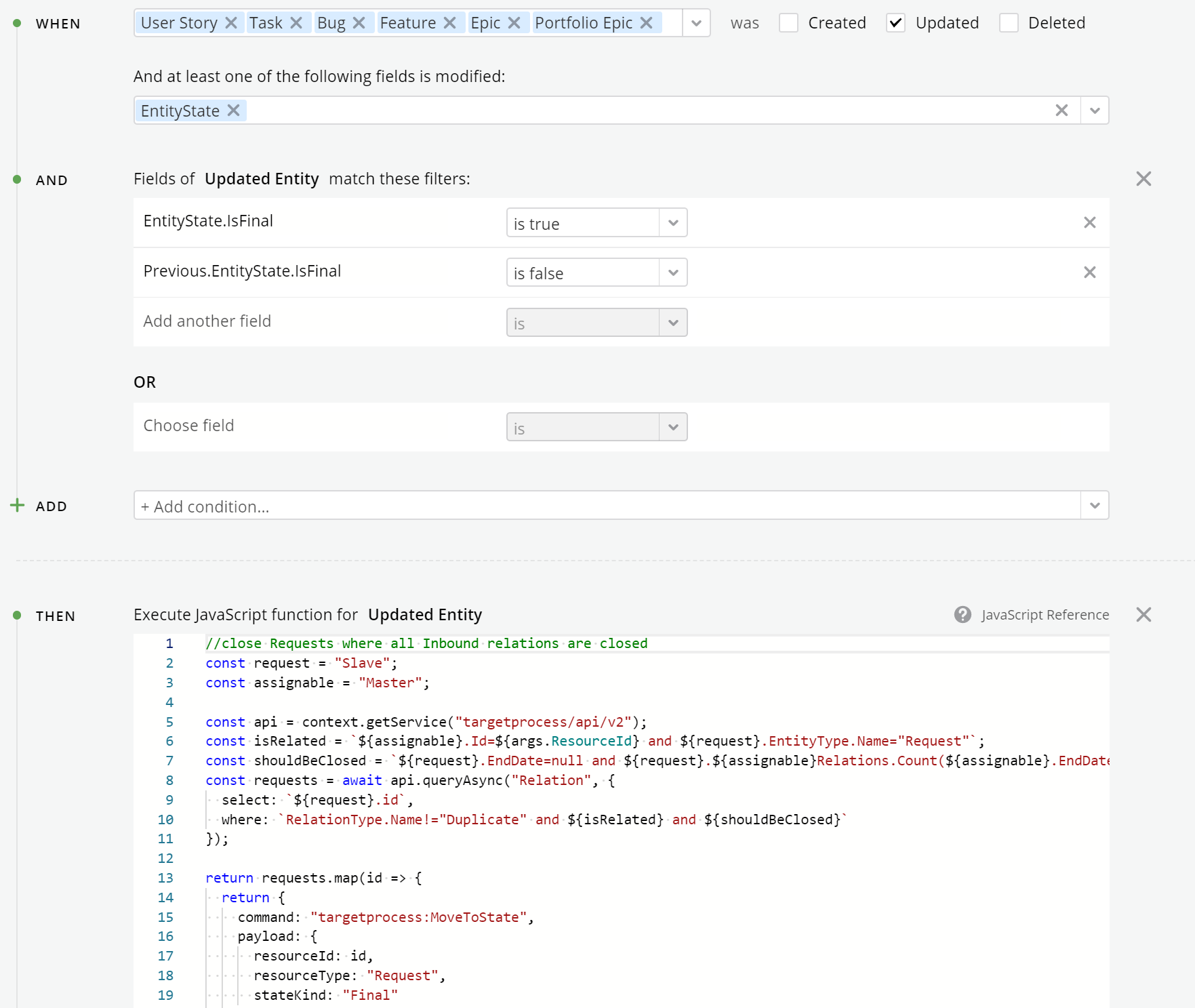
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory",
"Task",
"Bug",
"Feature",
"Epic",
"PortfolioEpic"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"EntityState"
]
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "is true"
}
},
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "field",
"name": "Previous",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": null,
"operator": {
"type": "is false"
}
}
]
}
]
},
{
"type": "action:JavaScript",
"script": "//close Requests where all Inbound relations are closed\nconst request = \"Slave\";\nconst assignable = \"Master\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst isRelated = `${assignable}.Id=${args.ResourceId} and ${request}.EntityType.Name=\"Request\"`;\nconst shouldBeClosed = `${request}.EndDate=null and ${request}.${assignable}Relations.Count(${assignable}.EndDate=null)=0`;\nconst requests = await api.queryAsync(\"Relation\", {\n select: `${request}.id`,\n where: `RelationType.Name!=\"Duplicate\" and ${isRelated} and ${shouldBeClosed}`\n});\n\nreturn requests.map(id => {\n return {\n command: \"targetprocess:MoveToState\",\n payload: {\n resourceId: id,\n resourceType: \"Request\",\n stateKind: \"Final\"\n }\n };\n})"
}
]
Close a Request when all related outbound entities are closed
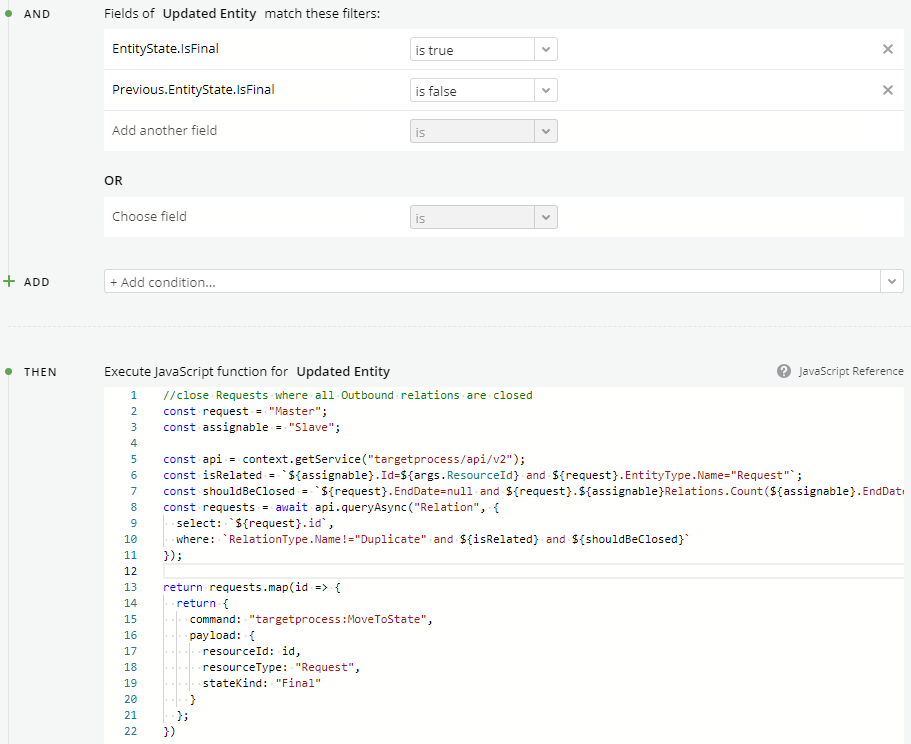
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory",
"Task",
"Bug",
"Feature",
"Epic",
"PortfolioEpic"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"EntityState"
]
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "is true"
}
},
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "field",
"name": "Previous",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": null,
"operator": {
"type": "is false"
}
}
]
}
]
},
{
"type": "action:JavaScript",
"script": "//close Requests where all Outbound relations are closed\nconst request = \"Master\";\nconst assignable = \"Slave\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst isRelated = `${assignable}.Id=${args.ResourceId} and ${request}.EntityType.Name=\"Request\"`;\nconst shouldBeClosed = `${request}.EndDate=null and ${request}.${assignable}Relations.Count(${assignable}.EndDate=null)=0`;\nconst requests = await api.queryAsync(\"Relation\", {\n select: `${request}.id`,\n where: `RelationType.Name!=\"Duplicate\" and ${isRelated} and ${shouldBeClosed}`\n});\n\nreturn requests.map(id => {\n return {\n command: \"targetprocess:MoveToState\",\n payload: {\n resourceId: id,\n resourceType: \"Request\",\n stateKind: \"Final\"\n }\n };\n})"
}
]
Reopen a request when a related outbound item is reopened
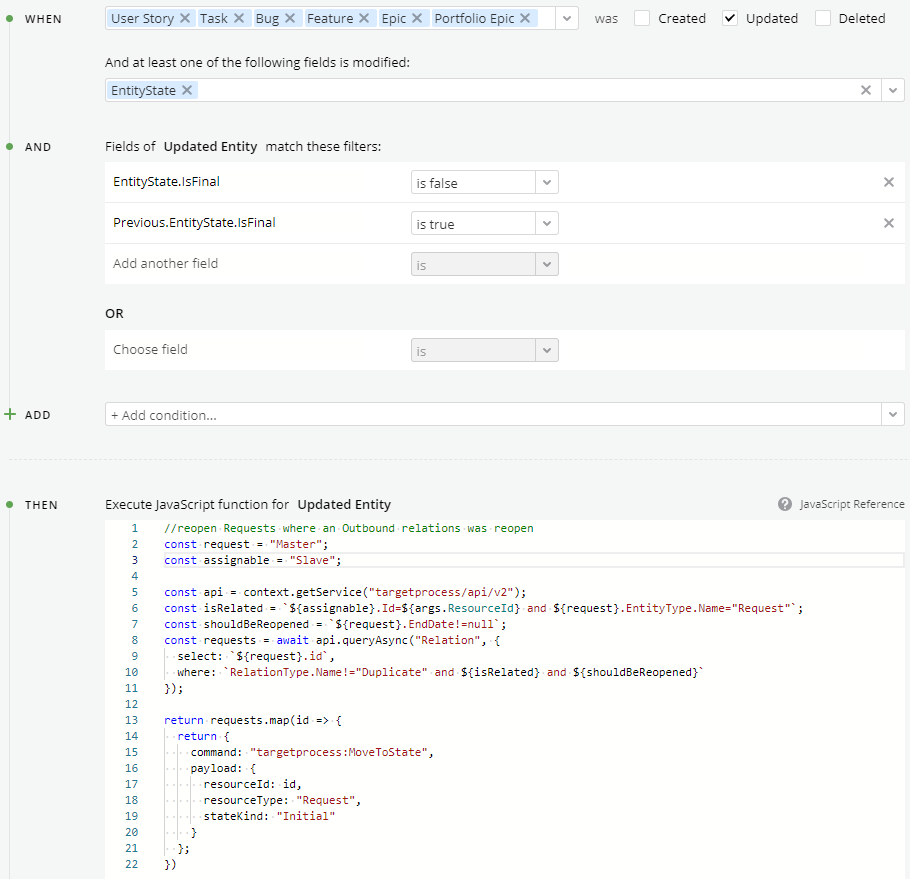
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory",
"Task",
"Bug",
"Feature",
"Epic",
"PortfolioEpic"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"EntityState"
]
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "is false"
}
},
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "field",
"name": "Previous",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": null,
"operator": {
"type": "is true"
}
}
]
}
]
},
{
"type": "action:JavaScript",
"script": "//reopen Requests where an Outbound relations was reopen\nconst request = \"Master\";\nconst assignable = \"Slave\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst isRelated = `${assignable}.Id=${args.ResourceId} and ${request}.EntityType.Name=\"Request\"`;\nconst shouldBeReopened = `${request}.EndDate!=null`;\nconst requests = await api.queryAsync(\"Relation\", {\n select: `${request}.id`,\n where: `RelationType.Name!=\"Duplicate\" and ${isRelated} and ${shouldBeReopened}`\n});\n\nreturn requests.map(id => {\n return {\n command: \"targetprocess:MoveToState\",\n payload: {\n resourceId: id,\n resourceType: \"Request\",\n stateKind: \"Initial\"\n }\n };\n})"
}
]
Reopen a request when a related inbound item is reopened
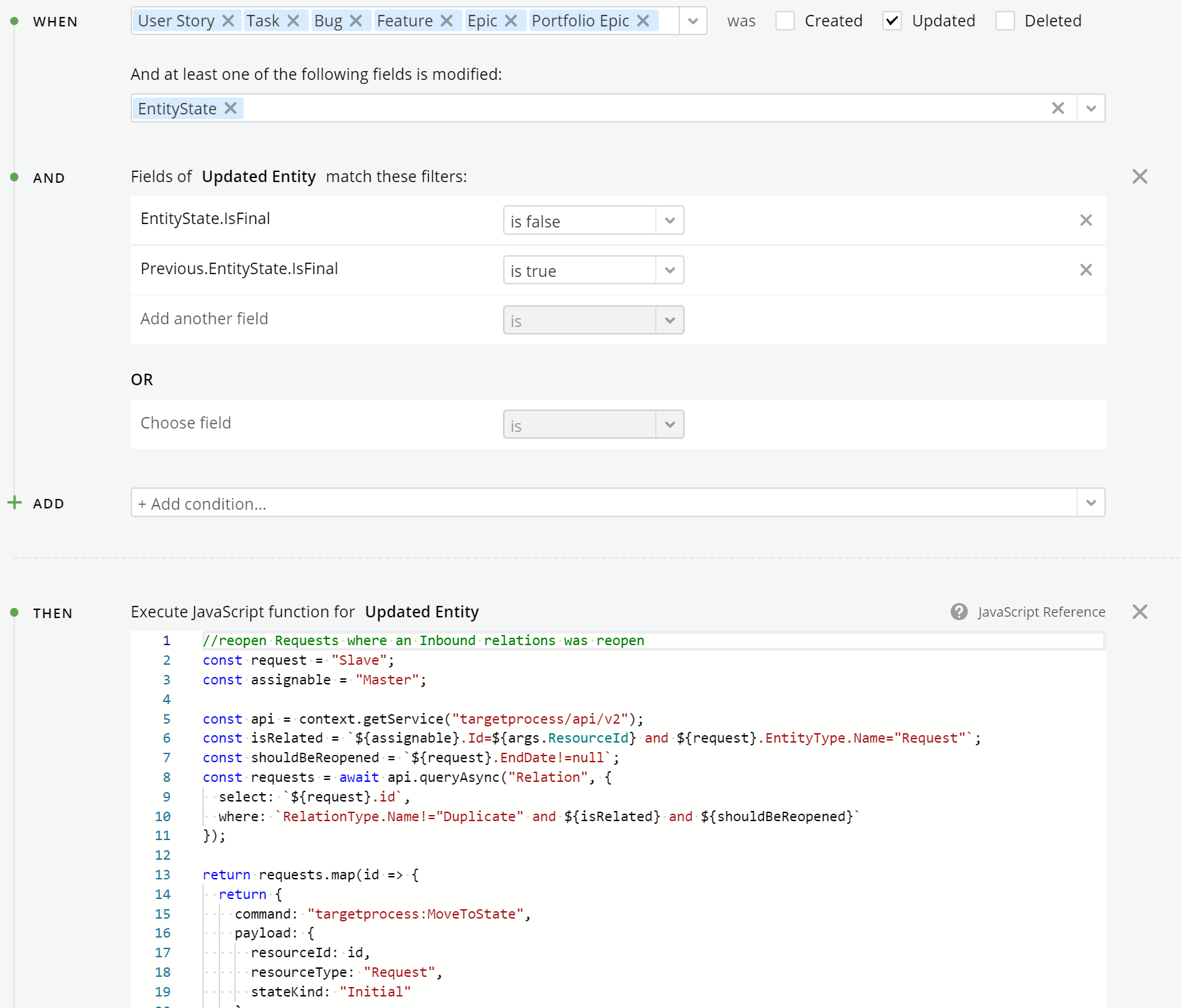
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory",
"Task",
"Bug",
"Feature",
"Epic",
"PortfolioEpic"
],
"modifications": {
"created": false,
"deleted": false,
"updated": [
"EntityState"
]
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "is false"
}
},
{
"target": {
"type": "field",
"name": "IsFinal",
"target": {
"type": "field",
"name": "EntityState",
"target": {
"type": "field",
"name": "Previous",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": null,
"operator": {
"type": "is true"
}
}
]
}
]
},
{
"type": "action:JavaScript",
"script": "//reopen Requests where an Inbound relations was reopen\nconst request = \"Slave\";\nconst assignable = \"Master\";\n\nconst api = context.getService(\"targetprocess/api/v2\");\nconst isRelated = `${assignable}.Id=${args.ResourceId} and ${request}.EntityType.Name=\"Request\"`;\nconst shouldBeReopened = `${request}.EndDate!=null`;\nconst requests = await api.queryAsync(\"Relation\", {\n select: `${request}.id`,\n where: `RelationType.Name!=\"Duplicate\" and ${isRelated} and ${shouldBeReopened}`\n});\n\nreturn requests.map(id => {\n return {\n command: \"targetprocess:MoveToState\",\n payload: {\n resourceId: id,\n resourceType: \"Request\",\n stateKind: \"Initial\"\n }\n };\n})"
}
]
Reopen a request when a new open outbound relation is added
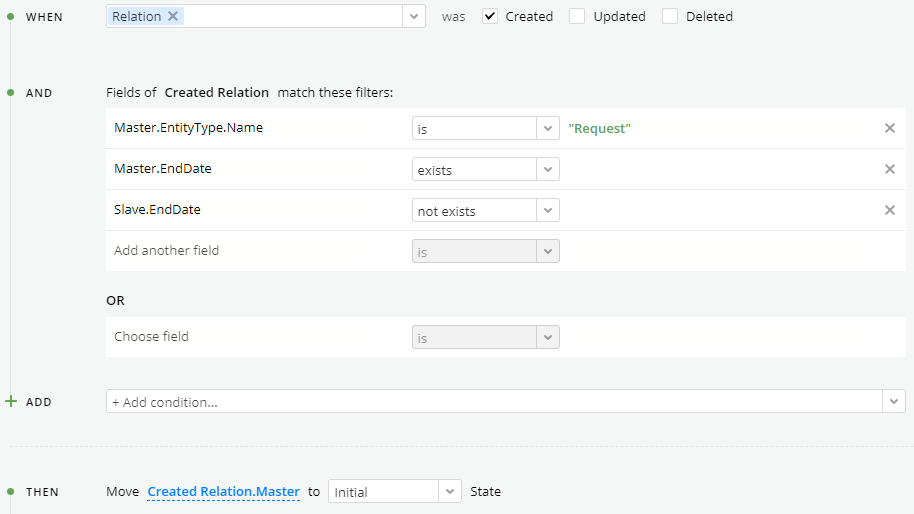
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Relation"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "Name",
"target": {
"type": "field",
"name": "EntityType",
"target": {
"type": "field",
"name": "Master",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": {
"type": "constant",
"value": "Request"
},
"operator": {
"type": "is"
}
},
{
"target": {
"type": "field",
"name": "EndDate",
"target": {
"type": "field",
"name": "Master",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "exists"
}
},
{
"target": {
"type": "field",
"name": "EndDate",
"target": {
"type": "field",
"name": "Slave",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "not exists"
}
}
]
}
]
},
{
"type": "action:targetprocess:MoveToState",
"stateKind": "Initial",
"target": {
"type": "field",
"name": "Master",
"target": {
"type": "pipelineBlockOutput"
}
}
}
]
Reopen a request when a new open inbound relation is added
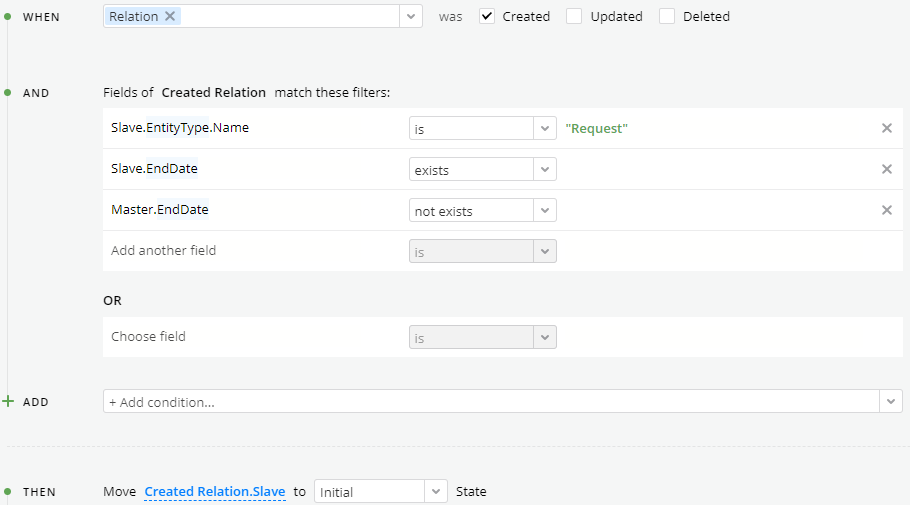
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"Relation"
],
"modifications": {
"created": true,
"deleted": false,
"updated": false
}
},
{
"type": "filter:Relational",
"or": [
{
"and": [
{
"target": {
"type": "field",
"name": "Name",
"target": {
"type": "field",
"name": "EntityType",
"target": {
"type": "field",
"name": "Slave",
"target": {
"type": "pipelineBlockOutput"
}
}
}
},
"value": {
"type": "constant",
"value": "Request"
},
"operator": {
"type": "is"
}
},
{
"target": {
"type": "field",
"name": "EndDate",
"target": {
"type": "field",
"name": "Slave",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "exists"
}
},
{
"target": {
"type": "field",
"name": "EndDate",
"target": {
"type": "field",
"name": "Master",
"target": {
"type": "pipelineBlockOutput"
}
}
},
"value": null,
"operator": {
"type": "not exists"
}
}
]
}
]
},
{
"type": "action:targetprocess:MoveToState",
"stateKind": "Initial",
"target": {
"type": "field",
"name": "Slave",
"target": {
"type": "pipelineBlockOutput"
}
}
}
]
Updated over 4 years ago