Example: When repositories custom field value changes → create branch/merge request
In this example, there is a 'Repos' drop-down custom field for User Story, Task, Bug or Feature, which lists repositories names. It must be exact name of the repository without parent subgroups.
Automation rule reacts on custom field update, and if the value is set to non-empty value, then branch and merge request will be created in selected repository.
In GitLab it will create a branch and a merge request, and they will be linked to the story. In Azure DevOps a branch and a pull request will be created.
In case of GitHub, Bitbucket only branch will be created, as pull requests cannot be created when no code changes committed.
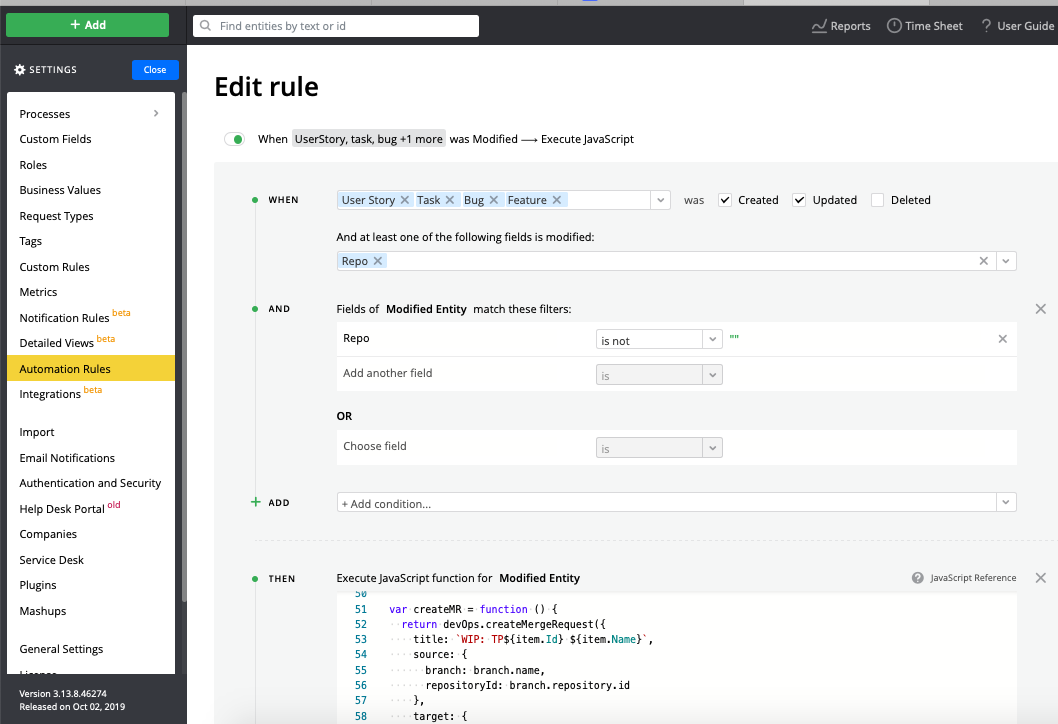
If you replicated WHEN clause, then copy and paste this THEN to rule:
const api = context.getService("targetprocess/api/v2")
const devOps = context.getService("devOps")
const item = args.Current
var repo = item["Repo"]
if (!repo) return [];
const branchName = `TP${item.Id}_${item.Name.replace(/\W+/g, '_')}`
console.log('Repo ', repo)
console.log('Creating branch ', branchName)
var branch = await devOps.createBranch(
{
name: branchName
},
repo
)
await devOps.createMergeRequest({
title: `WIP: TP${item.Id} ${item.Name}`,
source: {
branch: branch.name,
repositoryId: branch.repository.id
},
target: {
branch: "master",
repositoryId: branch.repository.id
}
})
return []
If the rule totally fits, you can copy it whole and paste to your rule. For this, switch rule editor to 'Raw json View' copy this code and paste into you rule in raw json editor mode:
[
{
"type": "source:targetprocess:EntityChanged",
"entityTypes": [
"UserStory",
"task",
"bug",
"feature"
],
"modifications": {
"created": true,
"deleted": false,
"updated": [
"Repo"
]
}
},
{
"or": [
{
"and": [
{
"value": {
"type": "constant",
"value": ""
},
"target": {
"name": "Repo",
"type": "field",
"target": {
"type": "pipelineBlockOutput"
}
},
"operator": {
"type": "is not"
}
}
]
}
],
"type": "filter:Relational"
},
{
"type": "action:JavaScript",
"script": "const api = context.getService(\"targetprocess/api/v2\")\nconst devOps = context.getService(\"devOps\")\nconst item = args.Current\n\nvar repo = item[\"Repo\"]\n\nif (!repo) return [];\n\nconst branchName = `TP${item.Id}_${item.Name.replace(/\\W+/g, '_')}`\n\nconsole.log('Repo ', repo)\nconsole.log('Creating branch ', branchName)\n\nvar branch = await devOps.createBranch(\n {\n name: branchName\n },\n repo\n)\n\nawait devOps.createMergeRequest({\n title: `WIP: TP${item.Id} ${item.Name}`,\n source: {\n branch: branch.name,\n repositoryId: branch.repository.id\n },\n target: {\n branch: \"master\",\n repositoryId: branch.repository.id\n }\n})\n\nreturn []"
}
]
Updated over 4 years ago